How to configure internationalization in Next.js
Learn the process of web application customisation (i18n) in Next.js redirects to create multilingual web applications. Learn the basics of global personalisation and its importance in today's digital landscape. Dive into implementing international redirects in Next.js, effectively managing different language versions. Learn how to define language paths, integrate with translation systems and optimise for SEO and accessibility. Ideal for developers and project managers who want to extend the reach of their Next.js application by providing an excellent user experience across languages and locations.
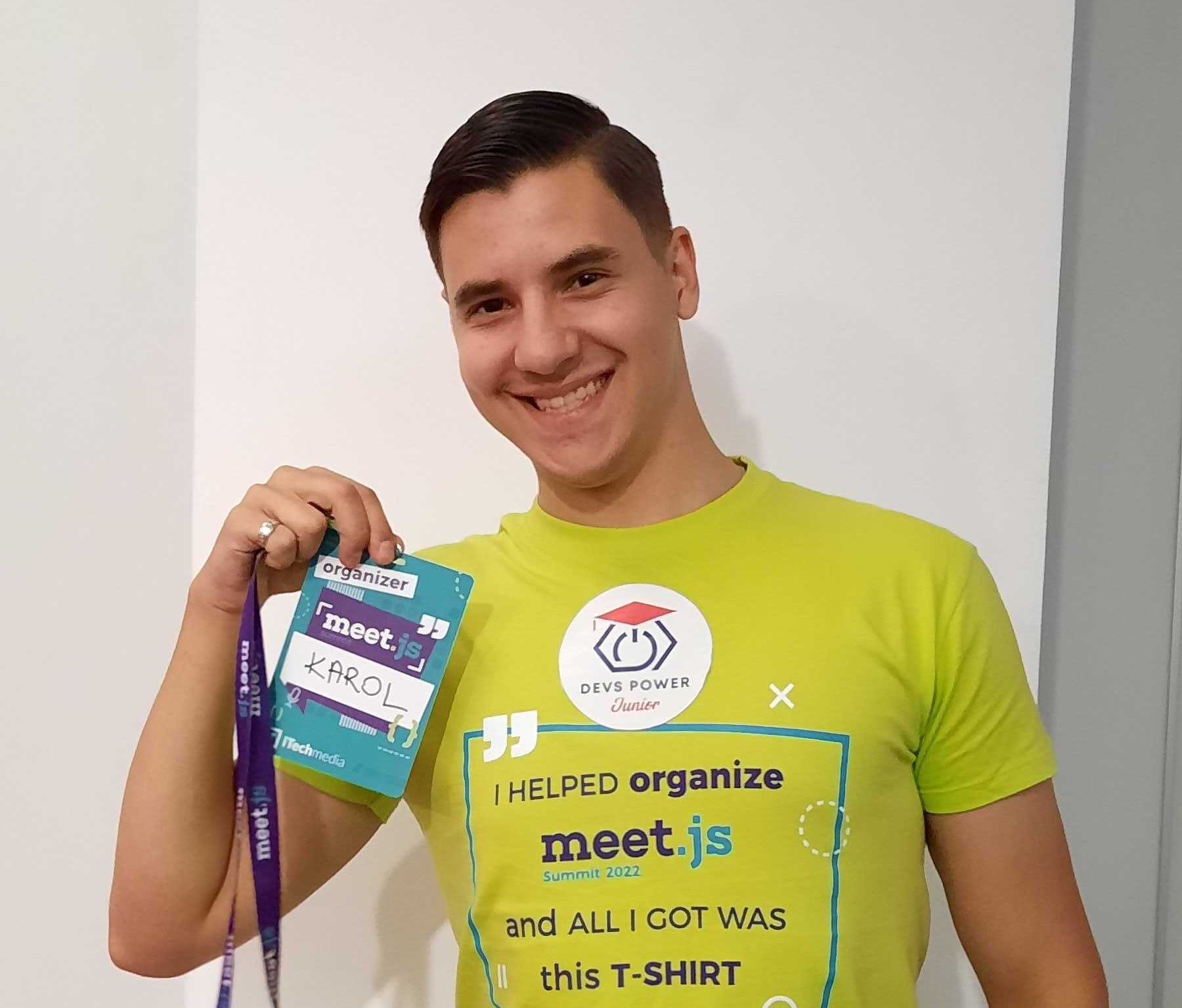
6th January 2024
In today's globally connected world, providing content in multiple languages has become not only an added value, but often a key element in the success of web applications. In this perspective, the concept of international customisation, also known as i18n, is emerging. It is a process that enables applications to effectively adapt to different languages and cultures, providing users around the world with a more personalised and accessible experience. One of the key elements of international customisation is international redirection, which is a mechanism for managing multilingual paths within an app. In this article, we will look at what exactly international redirection means and why it is so important in the context of creating modern global web applications.
International redirection, refers to the process of configuring a web application so that it can handle different URLs for different language versions. The term 'i18n' is an abbreviation for 'Internationalization', where the number 18 represents the number of omitted letters between the 'i' and the 'n'. Below are some key aspects of international redirection and their importance in creating modern global web applications:
Multi-language support: International redirection enables support for multiple languages in web applications, which is important in a world where users consume digital content in different languages.
Customised URLs: Each language version of the website can have a unique URL, making it easier for users to navigate and understand as the content is available in their language.
Automatic language recognition: Apps can automatically identify a user's preferred language based on browser settings or location, improving usability and accessibility.
SEO optimisation: Having separate URLs for different language versions can improve search engine positioning. Search engines can more easily index content in different languages as separate pages, increasing visibility in search results.
Maintaining status when changing languages: Good international redirection practices ensure that a language change does not reset the state of an application, such as a shopping cart or completed form.
Facilitating localisation: International redirection facilitates the process of localisation, i.e. adapting the app to specific markets, not only by translating content, but also by taking into account local customs and preferences.
Improving user experience: Providing content in the user's native language has a positive impact on the user's experience of interacting with the app, increasing engagement and satisfaction.
In the next part of the article, we will show step by step the process of implementing multilingual support in your Next.js applications using the i18n library.
Before starting, you should download the following libraries using npm or yarn.
1npm i next-i18next react-i18next i18next
In Next.js's implementation of i18n redirects, natural functionality support and flexibility are key aspects, allowing developers to freely manage multilingual variants of the application. To start this process, let's create a file named: next-i18next.config.js, in the root directory of the project where we define the available languages and the default language of the application. For example:
1module.exports = {
2 i18n: {
3 locales: ['en-US', 'de', 'es-ES'],
4 defaultLocale: 'en-US',
5 },
6}
Localisation strategies, such as subpath routing and domain routing, can be used in Next.js to create an application that supports multiple languages, catering for users from different regions of the world.
The next step is to wrap the main component of the application using the higher-order component "appWithTranslation":
1import { appWithTranslation } from 'next-i18next';
2
3const MyApp = ({ Component, pageProps }) => ( <Component {...pageProps} />);
4
5export default appWithTranslation(MyApp);
Then place the following function for each component placed in the pages folder. As the second argument, we pass the names of the files in the array for the serverSideTranslations function.
This array is actually the names of the json files that will be used by the server for translations on a given page when it is built. If the filename that we want to use on a given page for translations is not included in this array, the server will not be able to apply the translations, which will result in the page being rendered with keys instead of the correct content. This in turn will result in a degradation of SEO and user experience.
1import { serverSideTranslations } from 'next-i18next/serverSideTranslations'
2
3export async function getStaticProps({ locale }) {
4 return {
5 props: { ...(await serverSideTranslations(locale, ['common'])) }
6 }
7}
The next step is to integrate with a translation management library, which is react-i18next. This library allows translation files to be easily managed and used in React components. For example, using the useTranslation hookup, we can dynamically display texts in the selected language:
1import { useTranslation } from 'next-i18next';
2
3function MyComponent() {
4 const { t } = useTranslation('common');
5 return <p>{t('welcome_message')}</p>;
6}
Access to location information
In Next.js, information about a user's location can be used to present content in an appropriate language, tailored to their regional preferences. Here is how this information can be obtained and used: Using the Hook useRouter
In Next.js, the useRouter hook from the next/router library allows access to information about the user's current path and location. Example of use:
1import { useRouter } from 'next/router';
2
3function MyComponent() {
4 const router = useRouter();
5 const { locale, pathname } = router;
6// Use 'locale' to customize the content of the component
7}
In this example, locale contains the current language of the page, which can be used to display translations or customize content.
Transition between sites
Both next/link and next/router in Next.js can be used to manage transitions between locations in the context of internationalisation (i18n). Here is how both of these tools can be used to effectively switch languages in a Next.js application: Transition using next/link: The Link component from Next.js is great for creating navigation between pages that also change location. You can use the locale attribute in Link to indicate which language version you want to redirect the user to.
1import Link from 'next/link';
2
3function Navbar() {
4 return (
5 <nav>
6 <Link href="/" locale="en">
7 <a>English</a>
8 </Link>
9 <Link href="/" locale="fr">
10 <a>Français</a>
11 </Link>
12 {/* Additional links to other locales */}
13 </nav>
14 );
15}
In this situation, when the user clicks on the link, he or she will be redirected to the home page (/) in the chosen location (en for English, fr for French).
Transition using next/router: The useRouter hook in Next.js allows dynamic routing management, including location changes. The push or replace methods from this hook can be used to change locations programmatically. Example:
1import { useRouter } from 'next/router';
2
3function LanguageSwitcher() {
4 const router = useRouter();
5 const changeLanguage = (language) => {
6 const currentPath = router.pathname;
7 router.push(currentPath, currentPath, { locale: language });
8 };
9
10 return (
11 <div>
12 <button onClick={() => changeLanguage('en')}>English</button>
13 <button onClick={() => changeLanguage('fr')}>Français</button>
14 {/* Additional buttons for other language */}
15 </div>
16 );
17}
In this example, the changeLanguage function modifies the location to the user's choice (e.g. en for English, fr for French), while keeping the same path (currentPath).
Both approaches, next/link and next/router, are useful in different scenarios. The Link component is ideal for static navigation links, while useRouter is more flexible and allows you to manage locations dynamically, for example based on user interactions or other conditions. The key is to choose the right method depending on the needs and context of your Next.js application.
Dynamic Redirects
Dynamic redirects and getStaticProps in the i18n (internationalisation) context in Next.js create a powerful combination for building multilingual, statically generated websites. Using dynamic routes, it is easy to create language- or region-dependent paths, for example /en/products/[id] for English and /de/products/[id] for German. Each path can correspond to the same page structure, but with the content translated into the relevant language.
The getStaticProps function in this context is used to retrieve language-specific data at the page build stage. For example, you can use getStaticProps in combination with dynamic routes to load product content in different languages. getStaticProps can retrieve product information, including description and prices, depending on the language specified in the path.
This implementation ensures efficient page loading while maintaining support for multiple languages. It also allows the generation of separate URLs for different language versions, which is beneficial for SEO. In addition, by pre-rendering the content, users receive faster page loading times and a better overall experience.
Static Paths
Combining non-dynamic routes with getStaticProps makes it possible to create multilingual, statically generated web pages, where each route corresponds to a specific language version of the page. Unlike dynamic routes, non-dynamic routes are predefined, meaning that each language has its own explicit path, for example /en/about for English and /de/about for German.
The getStaticProps and getServerSideProps functions in this view are used to retrieve language-specific data at the page build stage. For example, in the case of the /about page, the getStaticProps or getServerSideProps functions can have different logic depending on the value of the locale parameter which determines which language version of the page you are on. For example, get article content from different sources depending on the language.
This method allows for simple content management and easy mapping of routes to the appropriate language files. It is particularly useful in scenarios where the structure of the page remains constant and only the content changes depending on the language. It also ensures efficient page loading through pre-rendering, as well as having a beneficial effect on SEO, as each language version of the page has a unique URL. Routing Subpathways in i18n Routing:
One of the most direct approaches to implementing localisation strategies in Next.js is to use subpath routing. This method allows you to create separate URLs for each supported language version. For example, the English-language version of your app could be accessible at /en and the French version at /fr. This method not only makes it easier for users to navigate, but also has a positive effect on SEO optimisation by allowing search engines to effectively index content in different languages.
In the configuration above, en-US, de and es-ES are available for redirection, and en-US is set as the default regional language. For example, in the case of the pages/users.js file, the above configuration allows access to the following addresses:
/users /de/users /es-es/users
Remember that regional defaults do not have to have a prefix, but they can.
Another strategy is to use different domains or subdomains for different language versions. For example, the English version of your site could be hosted on example.com , while the French version could be hosted on example.fr. This method provides a clear distinction between language versions and may be more intuitive for some users. However, it requires more complex configuration and may result in higher maintenance costs.
1module.exports = {
2 i18n: {
3 locales: ['en-US', 'de', 'nl-NL', 'nl-BE'],
4 defaultLocale: 'en-US',
5
6 domains: [
7 {
8 domain: 'website.com',
9 defaultLocale: 'en-US',
10 },
11 {
12 domain: 'website.de',
13 defaultLocale: 'de',
14 },
15 {
16 domain: 'website.nl',
17 defaultLocale: 'nl-NL',
18 // Also considering other locations that should be targeted at this domain
19 // As indicated by the following statement
20 locales: ['nl-BE'],
21 },
22 ],
23 },
24}
Using the above code, we will be able to use the following URLs in the case of pages/users.js:
- website.com/users
- website.de/users
- website.nl/users
- website.nl/nl-BE/users
Automatic Regional Settings Detection in Next.js:
Next.js offers a powerful feature that automatically recognises regional settings, making it much easier to deliver content in the user's preferred language. This feature, based on i18n routing, enables the application to automatically detect a user's preferred language based on their browser or system settings, and then redirect them to the appropriate language version of the page.
The usage example is relatively simple. In the next-i18next.config.js configuration file, i18n can be configured with automatic recognition of regional settings as follows:
1module.exports = {
2 i18n: {
3 locales: ['de', '', 'es'],
4 defaultLocale: 'en',
5 localeDetection: true
6 },
7}
In this example, the list of supported language codes is included in locales, defaultLocale specifies the default language, and setting localeDetection: true enables automatic language detection. When the user visits the app, Next.js automatically identifies the preferred language based on the browser settings and redirects the user to the appropriate language version, for example /fr for French. This simple yet powerful solution provides a seamless user experience, eliminating the need for manual language selection and improving the overall accessibility of content.
Disabling Automatic Regional Settings Detection in Next.js:
Although the automatic recognition of regional settings in Next.js is a very useful feature, in some cases it may be necessary to disable it. This may be needed, for example, when you want to give users full control over language selection, or when automatic recognition may not work properly due to specific client or server settings. In such situations, Next.js allows developers to easily disable this feature by changing the configuration in the next.config.js file.
In the next step, we will use keys to translate the text into the appropriate language.
1const { i18n } = require('./next-i18next.config');
2
3module.exports = {
4 i18n,
5}
Before using translations, we need to create translation files in public/locales/[lng], where lng is a specific language e.g. en, es etc. and we place translation files in these directories in json format e.g. common.json. The next step will use keys to translate the text into the appropriate language.
Example directory structure:
Example files:
- public/locales/en/common.json
1{ 2 "welcome_message": "Hello" 3}
- public/locales/es/common.json
1{ 2 "welcome_message": "Hola" 3}
- public/locales/de/common.json
1 2{ 3 "welcome_message": "Hallo" 4}
Conclusion
Next.js's i18n (internationalisation) implementation enables the creation of multilingual web applications that are accessible and useful to a global audience. Through easy integration with Next.js, developers can take advantage of dynamic routes and methods such as getStaticProps and getServerSideProps to effectively manage content in different languages. This approach not only improves the user experience by providing content in their preferred language, but also significantly impacts SEO as each language version is treated as a separate page.
Although the implementation of i18n in Next.js offers many benefits, it also comes with challenges, such as content management, synchronisation of changes across language versions and support for specific regional formats. It is also important to remember to customise paths and URLs for each language version, which is crucial for SEO effectiveness.
The i18n library in conjunction with Next.js is a powerful tool that, although it requires a certain level of planning and implementation, significantly increases the accessibility and usability of web applications internationally.
Newsletter
Sign up to the Newsletter to gain access to the latest information