How to Add API Route in Next.js
Next.js is a well-known React framework used by developers for web applications. This framework is widely used to create high-performance web applications. But did you know, with Next.js you can also write on the backend side? Yes, this framework makes it easy for developers to create and deploy API endpoints. In this blog post, we'll explore the ins and outs of adding an API route in Next.js.
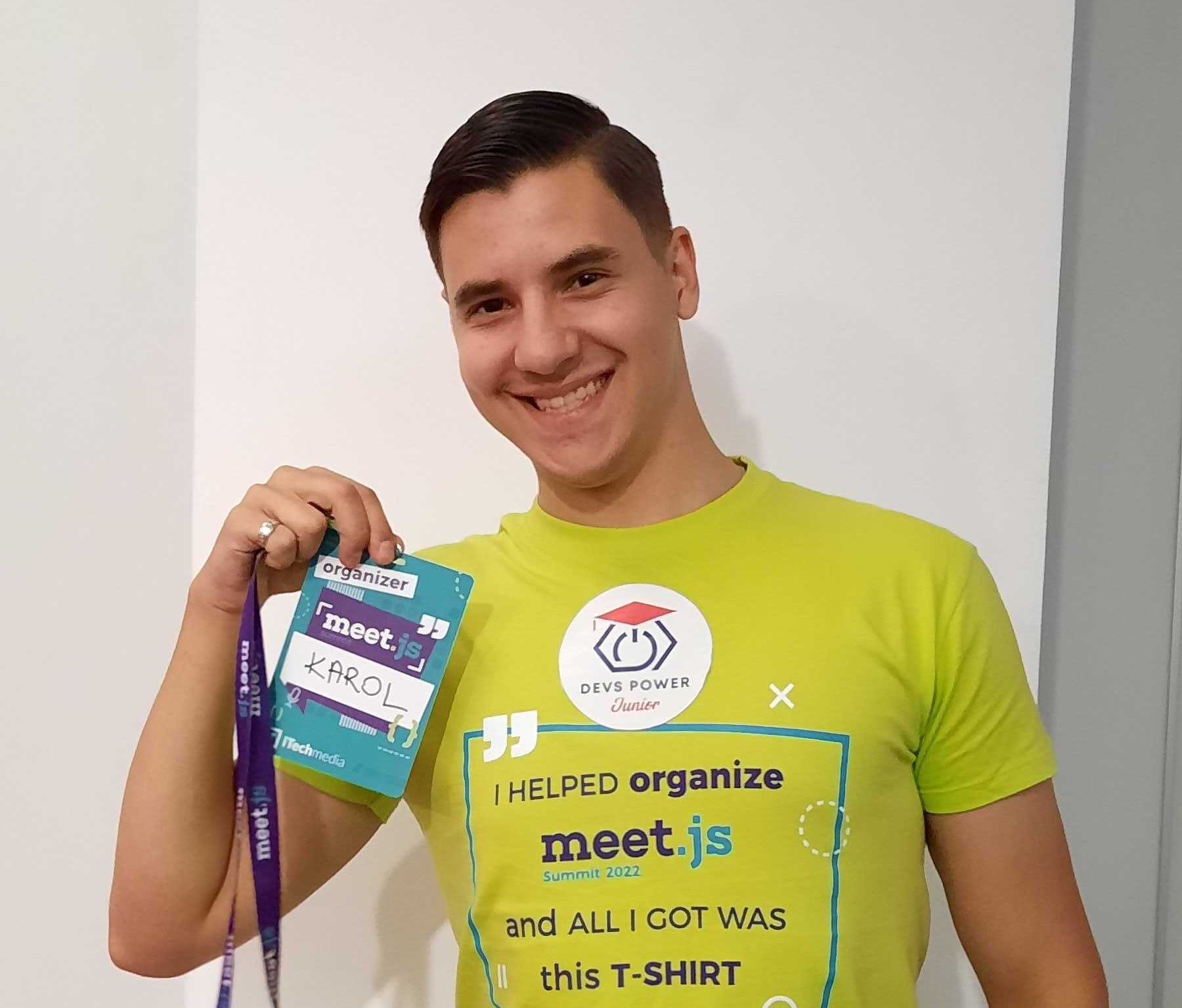
19th November 2023
Creating a New API Route
To create an API route in Next.js, you first need to create a new file in the directory ‘pages/api’. The file name will serve as the endpoint name and needs to end with the suffix .js. For instance, if you want to create an API endpoint named ‘users,’ the file name should be ‘users.js.’ Inside the file, you can add your endpoint logic, and Next.js will automatically route all incoming requests to that file.
Writing the Route Handler
When you browse to a new API route on the server-side, Next.js generates a new Node.js instance to handle the request. To write the route handler, you need to export a default Node.js function from the newly created file. The function should accept two arguments – a request object ‘req’ and a response object ‘res.’ Now, you can add your endpoint logic into the function. For example, if you want to send back a JSON response, you can use the response object and send data like this: res.status(200).json({message: “Hello World!”});
1export default function handler(req, res) {
2 res.status(200).json({ message: 'Hello World!' })
3}
This code is a simple example of an API handler in Next.js.
The handler function is the main handler function for this endpoint, taking two arguments: req (request) and res (response).
Inside the handler function, you can define the logic for this endpoint. In the example code, we return a response with a status of 200 (indicating success) and a JSON with the message "Hello World!".
Downloading data from the database
If you want to retrieve data from a database, you can do so using any Node.js database library or Next.js route API methods. Next.js provides two methods for retrieving data from API routes: getServerSideProps and getStaticProps. getServerSideProps retrieves data on the server side at request time, while getStaticProps retrieves data at compile time.
1export default function handler(req, res) {
2 const requestMethod = req.method;
3 switch (requestMethod) {
4 case 'GET':
5 res.status(200).json({ message: 'Hello from the GET API!' });
6 break;
7 case 'POST':
8 const body = req.body;
9 res.status(200).json({ message: `Hello from the POST API! You submitted the following data: ${body}` });
10 break;
11
12 // handle other HTTP methods
13 default:
14 res.status(405).json({ error: 'Method Not Allowed' });
15 }
16}
The handler function is the main handler function of this endpoint API, taking two arguments: req (request) and res (response). Inside this function, a switch statement is used to distinguish between the HTTP method passed in the request (whether it is GET, POST or another method). For the GET method, the code returns a response with a status of 200 with the message "Hello from GET API!". For the POST method, the code retrieves data from the request body (req.body) and returns a response with a status of 200 with a message that contains that data. If the request is an HTTP method other than GET or POST, the code returns a response with a status of 405 (Method not allowed), meaning that other methods are not supported by this endpoint. This example shows how the behaviour of the API can be adjusted depending on the HTTP method passed in the request, which is useful for creating versatile APIs that support different types of operations.
1
export async function getServerSideProps() {
2 const res = await fetch(`https://.../data`)
3 const data = await res.json()
4
5 return { props: { data } }
6}
7
8export default function Page({ data }) {
9 // Render data...
10}
This code shows how to use the getServerSideProps function in Next.js to retrieve server data and pass it to the page component. The getServerSideProps function is used in Next.js to generate data on the server during each page request. In this case, it is used to retrieve data from an external source (using fetch) and then pass this data as properties (props) to the Page component. The Page component takes this data as an argument and can render or use it to build the page content.
1
export default function Blog({ posts }) {
2 return (
3 <ul>
4 {posts.map((post) => (
5 <li>{post.title}</li>
6 ))}
7 </ul>
8 )
9}
10
11export async function getStaticProps() {
12 const res = await fetch('https://.../posts')
13 const posts = await res.json()
14
15 return {
16 props: {
17 posts,
18 },
19 }
20}
This code demonstrates the use of the getStaticProps function in Next.js to generate static data during page build. The Blog component is responsible for rendering the list of posts that will be retrieved during the page build. Inside the getStaticProps function, the data is retrieved from an external source, using fetch. The data is then parsed as JSON and passed as a property (props) to the Blog component. The getStaticProps function is called when the page is built on the server and is not executed on the client side. Thus, you can even make direct queries to the database when generating static page content.
How does the Api Route come to Vercel?
The Vercel platform automatically analyses the project structure, and then configures a runtime environment that can support API routes. While traditional web applications may require a server, Vercel allows you to serve pages and APIs without having to maintain a separate server.
In short, when you create an API route on Vercel and then deploy your project, Vercel automatically configures the infrastructure to support API routes. When someone sends an HTTP request to one of the API endpoints, Vercel automatically redirects that request to the appropriate file in the api folder, where it is handled according to the code written for that endpoint.
What is serverless and how do these serverless endpoints work?
Vercel offers a serverless approach to hosting, eliminating the need to personally manage the server infrastructure. Unlike classic hosting methods, where you are required to configure and maintain the server, Vercel takes on these tasks. As a result, you can concentrate your efforts on developing your application code. Not only does this model make things easier, but it also gives you the flexibility to scale your application according to its current load, meaning you only pay for the resources you actually use. When working with applications in Next.js, Vercel simplifies the process of creating and managing serverless endpoints. By automatically generating endpoints for API functions, Vercel provides seamless integration with Next.js, making it much simpler and more efficient to develop and manage applications in this environment.
CONTACT US
Do you have any question?
Tailored solutions are no secret to us. If you need a trustworthy partner - email us!